JSON Feed Validator
Use this validator to check if your JSON is valid and visible to our server.
For more info on this API, check out the specification.
slug
key is required.Array
(
[version] => https://jsonfeed.org/version/1
[title] => JWITHY
[description] => Feed for JWITHY
[home_page_url] => https://www.jwithy.com
[feed_url] => https://www.jwithy.com/jsonfeed.json
[items] => Array
(
[0] => Array
(
[id] => https://www.jwithy.com/my-heads-on-fire
[slug] => my-heads-on-fire-and-high-esteem
[title] => My head's on fire and high esteem
[summary] => I realized today that I was feeling that sort of anxiety that I often think of (and sometimes call out loud) as itchy. And the weird direction it
[content_html] => I realized today that I was feeling that sort of anxiety that I often think of (and sometimes call out loud) as itchy. And the weird direction it took me was “hey I should go see what omg.lol is up to,” and that turned into “I think I need to write a blog post.”
This never happens, so here we are.
I’m moving back to Portland in less than a week. The apartment I got is giving me all sorts of worries about money — it’s so beautiful, and so expensive — and I’m also trying (failing) to not lead with that when I want to tell people about its wonderful patio, building amenities, and location.
But more than that, and maybe more importantly, the process of moving this time has been a solo endeavor in a way it hasn’t ever before. I don’t have a partner to help, and most of my friends live in Portland — that’s why I’m heading back there, after a 5-year hiatus in Washington State — and so all the tasks are falling to me, with support from friends, but not help on the ground from them.
It’s mostly been ok! I stressed out one of my close buds, particularly on one of my panicked days — we’re working through that — but I’m feeling stronger, more capable, more prepared that I have maybe ever?
And yet.
The task of moving really taxes me. My ADHD brain loves to throw, “Really? We are working on more of this shit?” at me. The anxious part of me wants to know why I’m not doing even more, and the therapy-having part of me keeps telling me I’m going to fuck it all up.
But again, one thing that’s really hopeful (and helpful!): all of that stuff has usually been at a volume of maybe 2 out of 10 at most (except for the aforementioned day when it hit 11).
I can’t wait to return to Portland, to walking for coffee and taking a quick bus to see music. To being able to see friends all the time, instead of during sporadic visits. To knowing all the bus routes (still!) and the places that I’ve loved, and even the new ones that sound amazing.
But the way my mind works, I won’t truly realize that’s what’s at the end of this journey until maybe the day before the move, or maybe when I drive away from Lakewood, from the shared life I had yet no longer have. I find it incredibly tough to be excited about a trip when I’m packing for a trip…and this is the biggest trip I’ve taken since I left Portland in 2020.
I’m scared, I’m tired, I’m getting there, I’m strong, I’m excited, I’m sad, I’m so much right now.
I’m ready.
If you know how to contact me elsewhere, and you’re the kind of person who sends mail, shoot me a text / email / whatever and I’ll send my address along. I’d love to have sweet messages waiting for me when I arrive.
[date_published] => 2025-02-10T00:00:00-08:00
[url] => https://www.jwithy.com/my-heads-on-fire
)
[1] => Array
(
[id] => https://www.jwithy.com/it-has-been-a-time
[slug] => it-has-been-a-time
[title] => It Has Been a Time
[summary] => Dear Readers, It has been a time. I got to go the final XOXO. It felt like a found family reunion — for me, and for many folks who had attended
[content_html] => Dear Readers,
It has been a time.
I got to go the final XOXO. It felt like a found family reunion — for me, and for many folks who had attended before this year. I got to see Open Mike Eagle sing “Birdhouse in Your Soul (<–video link) at our very own karaoke. I saw both Never Post and Annie from Depths of Wikipedia bring the house down during a glorious night of performances.
And I did that after battling COVID — it didn’t hit me all that hard, physically. Mentally? I was, of course, a wreck. I took my antivirals to hopefully ease the long term pain, and the doctor cleared me for the conference.
I’ve also had some really exciting, promising interviews…and one pretty rough one. 😂 I’ve hit some lows a bit, again, but also bounced back. I’ve gotten incredibly excited about different web projects, heard about (and hell yeah-ed about) new css that’s coming soon, and considered how to create my own css crimes. And I’ve also looked around and gone, “wait, it’s been almost two months since I was fired up and working on my new site using eleventy?!?!?”
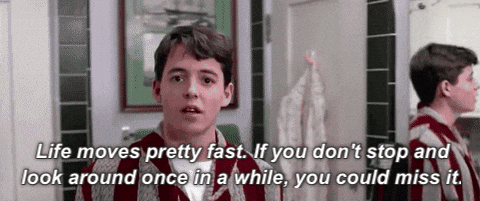
Anyway, because most folks likely won’t see this yet, here’s a logo I built in CSS for a meetup that doesn’t exist / I haven’t started yet:
See the Pen tacoma-logo-rubik by Jim Withington (@jwithington) on CodePen.
It’s a time of highs and lows, y’all. 🎢
[date_published] => 2024-09-04T00:00:00-07:00
[url] => https://www.jwithy.com/it-has-been-a-time
)
[2] => Array
(
[id] => https://www.jwithy.com/conferencing-talking-learning
[slug] => conferencing-talking-learning
[title] => Conferencing, Talking, Learning
[summary] => I just submitted a talk for SquiggleConf, a conference about web dev tooling. In a way that, to me, feels very like me, it’s not about electronic
[content_html] => I just submitted a talk for SquiggleConf, a conference about web dev tooling. In a way that, to me, feels very like me, it’s not about electronic tools at all.
I submitted a talk about intuition as your best tool.
One of the luckiest, best decisions I made in 2020 was signing up for Jocelyn K. Glei’s Hi-Fi course, a course that, at that time, was something like 5 or 6 months long, running from (I believe) around April 2020 until September or so.
Those months were…interesting, if you recall.
But the class? All about intuition.
About tapping into something deeper than the surface. Things lieke:
- intuitively picking images for a collage at the beginning of the course, not even knowing what the collage “meant” yet
- meditation and breathing techniques
- paying real attention to the “inputs” we take in (music, podcasts, news, etc.)
- using tarot cards for insight
It wasn’t all woo-woo stuff, though I have to say, the woo-woo stuff really helped.
It left a mark on me, and when I’m struggling, it feels a bit like I’ve lost my way as far as my intuition.
So, for a conference like SquiggleConf that will likely have a lot to say about productivity tools, new AI stuff, and VS Code extensions, I of course thought, “What if the best tool is your own gut feeling?”
We’ll see if the talk gets accepted — but I’m pretty sure I’ll be submitting it elsewhere, either way. 😀
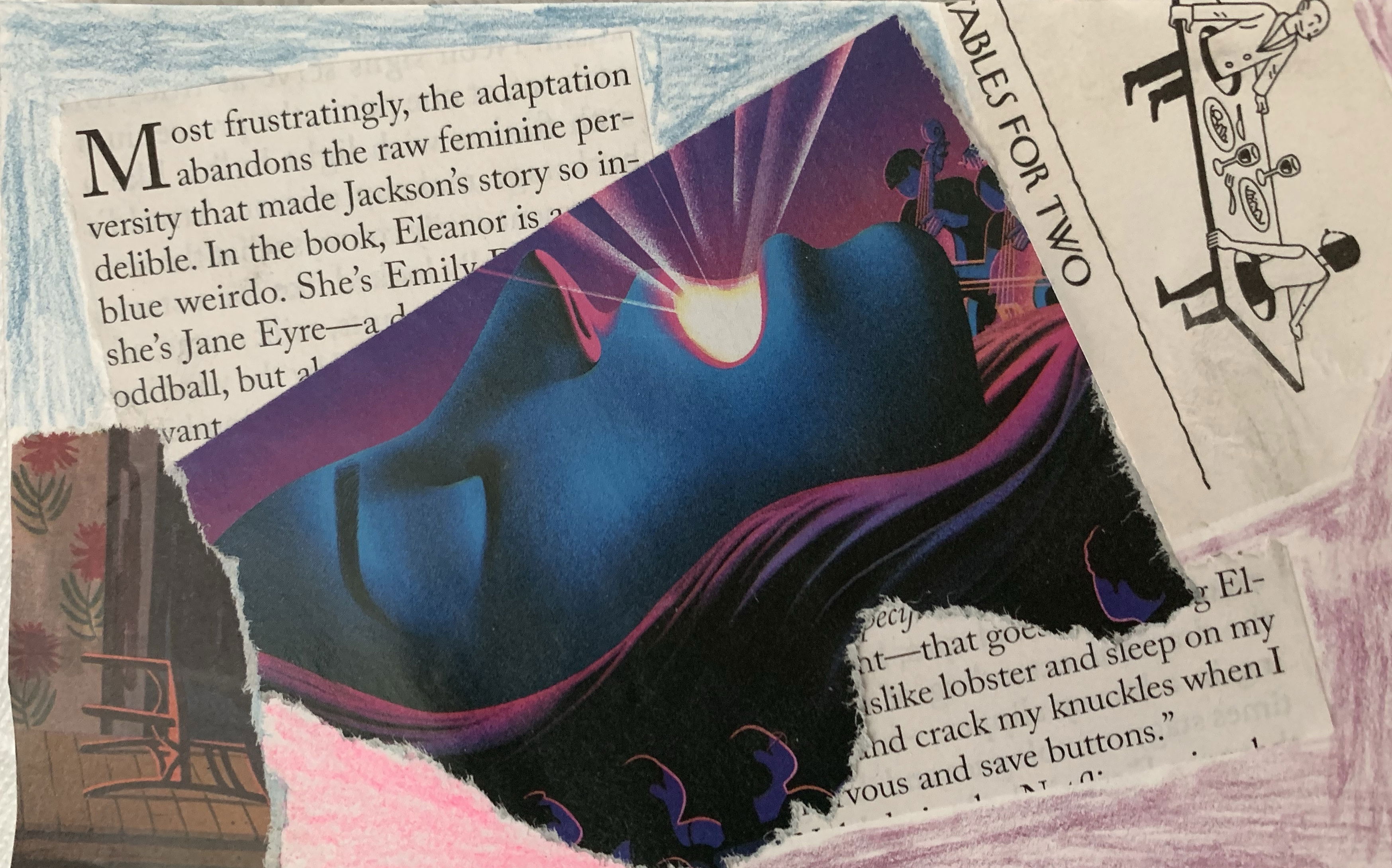
[date_published] => 2024-07-11T00:00:00-07:00
[url] => https://www.jwithy.com/conferencing-talking-learning
)
[3] => Array
(
[id] => https://www.jwithy.com/ignore-it
[slug] => ignore-it-then-deploy-it
[title] => Ignore It, then Deploy It
[summary] => Today I realized I wanted to set this thing up for real continous deployment — I no longer wanted to have to drag and drop to deploy the thing. I
[content_html] => Today I realized I wanted to set this thing up for real continous deployment — I no longer wanted to have to drag and drop to deploy the thing. I like using git even for personal projects, so it’s a no-brainer to make it so that pushing to remote causes a deploy.
I set it up on Netlify kinda by default, simply because it was in the eleventy tutorial’s deployment section, and I gotta say: it’s nice to be back! I used to play around with Netlify a lot in the past, but haven’t had a need to for a long time (as I was using surge.sh, Github Pages, or Blot, depending on what I was working on).
And those are all good tools for what they are! But with Eleventy, I’m trying to do it The Eleventy Way™️, and so using the first thing they mentioned in the tutorial felt right. 😀
A tiny gitignore explainer
I also knew that I never want to throw a git repo on GitHub without creating a .gitignore
file. For the uninitiated, that’s a file that tells git, “hey, don’t ever include these files in the repo.” Briefly speaking, this prevents you from checking in:
- files that don’t need to be in your repo and that take up a bunch of space, like your
nodemodules
folder
- stuff that operating systems or apps use that have nothing to do with your project, like VS Code settings or all the junk that macOS dumps into folders
- most importantly: app secrets, environmental variables, and anything else that could result in something terrible like your API getting published for anyone to grab and use
That last one is a huge gotcha, and a bit of the reason why I use deployment services in the first place: people have built deploy tools that mean I’m not working too close to the metal. I like safeguards that prevent me from getting a giant web hosting bill, don’t you?
Let’s build that file
As a front end dev I’m absolutely well-versed in using a git ignore file, but I’m rarely the originator of it. At my last job, we inherited a lot of ignore files since our app began with us forking Backstage.
So this time, it ’s my turn!
The Eleventy docs weren’t super helpful, and neither was the actual Eleventy .gitignore
file, since it…had a lot of stuff that felt very specific to the project, not to what a user like me would need.
After some Googling, I ended up finding a couple of sources for my main tools: VS Code, on a Mac. I also found someone’s basic boilerplate version and deleted ths stuff I figured I wouldn’t use. I kept the sources in the file, both for me, and so I can credit them. You can see the file in the now-published GitHub repo. Hooray for tools built by folks!
A non-zero amount of what’s in that file represents things I haven’t built yet, like environmental variables and such. To me, that makes it a good safeguard — it’s already there when I do need it, and it prevents my “go go go” hyperfocus brain from missing the important stuff later.
Time to deploy
After I’m sure I have that all ready to go, it’s time to deploy. I used the GitHub CLI to push the local folder to a GitHub repo. From there, I followed the defaults in Netlify, and I did run into one hiccup: because I’m using eleventy 3.0, the default eleventy
build command failed.
I changed it to npx @11ty/eleventy@canary
, which is the local build command, and now it’s all up and running! 🥳
[date_published] => 2024-07-04T00:00:00-07:00
[url] => https://www.jwithy.com/ignore-it
)
[4] => Array
(
[id] => https://www.jwithy.com/accessibility-notes
[slug] => accessibility-notes
[title] => Accessibility Notes
[summary] => One thing that I believe in strongly: accessibility needs to be part of any project from the start, not just afterwards. Today I ran some Deque axe
[image] => https://www.jwithy.comhttps://cdn.blot.im/blog_03baca9562d34d128abe1318c3d12c5a/_thumbnails/500c6f0b-b2d7-45e7-9cd2-a791f1af5116/large.png
[content_html] => One thing that I believe in strongly: accessibility needs to be part of any project from the start, not just afterwards.
Today I ran some Deque axe tests on the day one stuff I posted the other day, and it turns out I already caught some issues:
- I made the
content for the site “Jim Withington,” but that header actually doesn’t describe what follows. This site will eventually be my portfolio/blog site, so even “Jim Withington’s Site” would be more appropriate.
- I didn’t have a language attribute in the main html tag.
- My links are marked as
’s. I know better than that. A huuuge rule of accessibility: elements should not be used for presentation. To be honest, I think this resulted from me using a tutorial, and I also don’t think of this “design” as something I’m going to keep 😂 However, again, a11y from the start!
- I never checked the color contrast the other day…and, obviously, even though I used placeholder colors, the grey and pink are not contrasting enough.(see below) So I’ll probably go back to using my fave
deeppink
until further notice as the background color. 😂

So today, those issues were the first things I worked on! Anything else could wait.
That said, once I did all that, I decided I wanted to quit having the full content of each post show up on the homepage. Even this early in the game, that bugged me. I’m working on adding a new variable called “teaser” where I can pull out a single line, add it to the front matter, and use that to represent the post. But it’s getting late and I have other stuff I want to do, so…tomorrow!
progress pic for today:
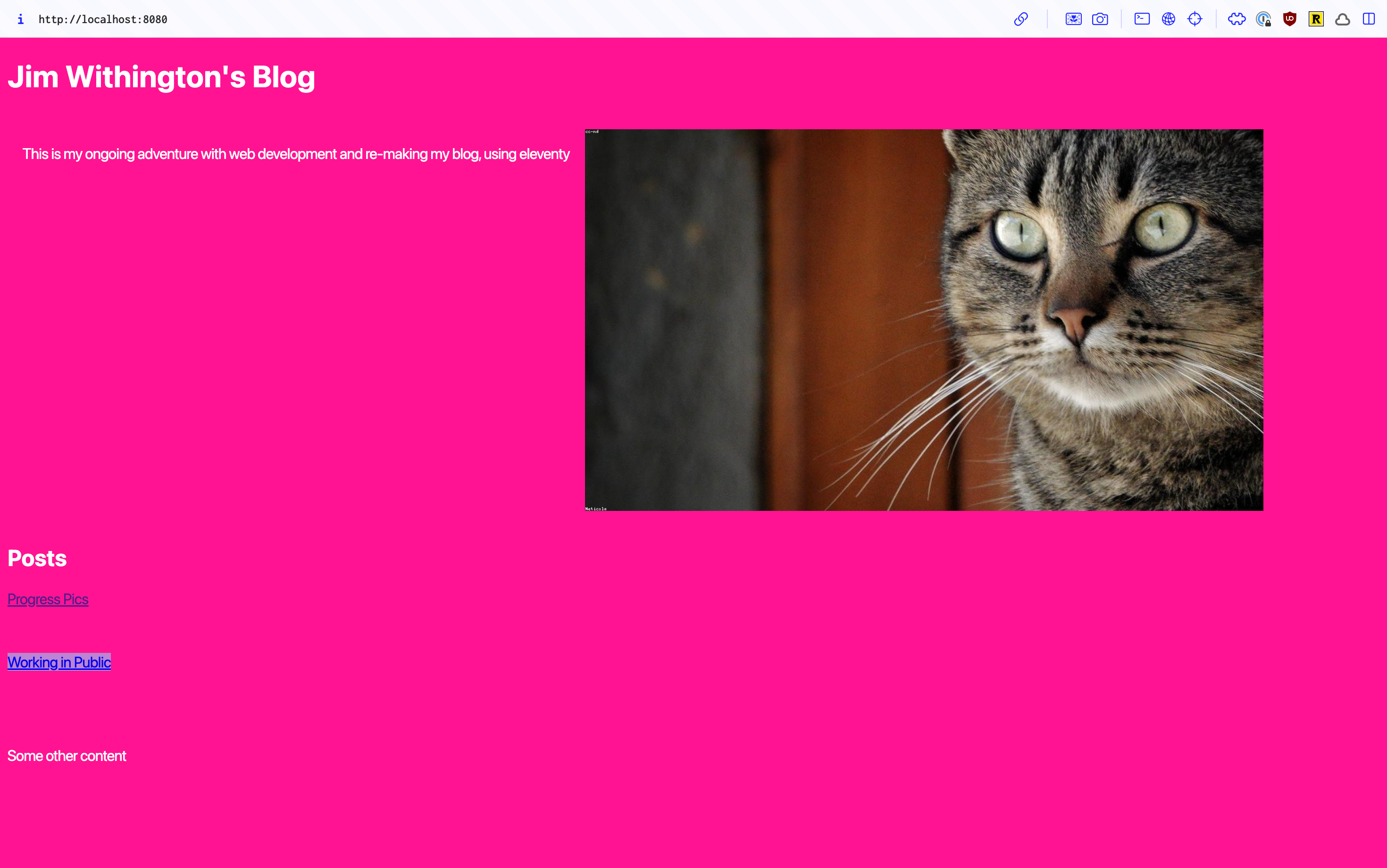
[date_published] => 2024-07-01T00:00:00-07:00
[url] => https://www.jwithy.com/accessibility-notes
)
[5] => Array
(
[id] => https://www.jwithy.com/working-in-public
[slug] => working-in-public
[title] => Working in Public
[summary] => I’m currently unemployed, and one thing that that means is time. I’ve got a lot of it. And I’m also a front end developer that misses doing the
[content_html] => I’m currently unemployed, and one thing that that means is time. I’ve got a lot of it.
And I’m also a front end developer that misses doing the fun-for-me parts of web development.
And I also just attended the wonderful CascadiaJS conference, where my head got filled up with so much learning with so many fun people.
For about a week now, I’ve had this itch. I want to blog again. I want to make it a semi-regular practice, something like what so many of my fellow omg.lol folks talk about when they link to barry’s post. I think Chris Coyier wrote about this, too.
At the conference, I also discovered something: my main jimwithington.com site’s CSS was borked. Totally busted. And that makes sense, as it was a template site I built before I knew how to do anything, and then I hosted it on surge.sh, and then I always said to myself “hmm, I should update that.”
(this kind of thing happens! as a web dev you don’t always want to do this stuff after work hours, too!)
And theeeennnnn I considered maybe doing a process where I set up a new blog with a new blog service, then eventually replace it with…whatever I come up with.
After a bunch of that, I realized some things:
- I have both website-from-scratch knowledge and stuff like, yknow, this here weblog space I’m not using.
- I’ve been wanting to try eleventy for a long time.
- Building something would both keep me from getting too rusty with my skills and hopefully provide me with talking points, stuff to show off, and new skills.
- Instead of worrying that my new thing sucks, I could also document the process, doing the “work in public” thing that I’m even more interested in after Jason’s Cascadia Talk.
I’m especially excited about that last point, and feeling a lot of momentum around it. I’m a huge believer in using personal sites as playgrounds to try out new things.
And Jason’s talk taught me that, hey, if you have stuff you love, and you do it in public, that’s a really good way for folks to then ask you to do that kinda work for them.
So let’s see how it goes!
For the most part, my posts are going to live at my weblog.lol, because I haven’t really been using that site, so I figured that now’s the time! When I finish the new thing, I’ll probably roll one of my existing sites (probably this one!) into the weblog one and then go from there.
Anyway, here’s the very first version of what I’m building. I can’t wait to keep adding to it and making it real!
[date_published] => 2024-06-29T00:00:00-07:00
[url] => https://www.jwithy.com/working-in-public
)
[6] => Array
(
[id] => https://www.jwithy.com/promising-design-patterns
[slug] => promising-design-patterns
[title] => Promising Design Patterns
[summary] => I talked to someone this evening about what I know and what I don’t know as far as web dev, React, JavaScript, and the like. And I had to admit a
[content_html] => I talked to someone this evening about what I know and what I don’t know as far as web dev, React, JavaScript, and the like.
And I had to admit a couple of things, right then and there:
- I only have a vague sense of what promises are (in JavaScript! in life, I’m good at keeping them).
- I can visualize what algorithms and design patterns are, but I have no idea if I know any of them offhand.
So I figured: why not learn something tonight?
And then I can post it! Bonus!
Promises
The thing that I knew, in the moment (probably due to the name? It’s right there on the tin): promises are a way to deal with asynchronicity.
In plain english: Once this other thing is done, then do this thing.
In fact, it literally uses then
to make the promise. What could be more readable?
This isn’t the only way to do this (see also: callbacks), but it does remove some complexity, in favor of more readable code.
On that linked example, I feel like the “single-use guarantee” portion is super important:
A promise only ever has one of three states at any given time:
- pending
- fulfilled (resolved)
- rejected (error)
A pending promise can only ever lead to either a fulfilled state or a rejected state once and only once, which can avoid some pretty complex error scenarios.
For the next post in that linked tutorial the author shows how to use promises in order to implement the fetch()
api. It makes so much sense, and is so readable:
fetch(this.getApiUrl())
.then(resp => resp.json())
.then(resp => {
const currentTime = resp.dateString;
this.setState({currentTime})
})
Even for someone who’s new to Promises, this makes so much sense. Give me the response, turn it into JSON
, and use it to set the current time.
Woop! We just learned about promises.
As far as algorithms and design patterns? Those are less of a quick study. I did, however, find a pretty good React-oriented design patters site here:
I even saw some that I use all the time, like the spread operator in JSX and Style Components, or have learned about already, like Event Switches. Some of this feels like “unknown knowns,” as it were.
I’ve definitely got some more resources to add the list now — I’m glad I had this convo today, and took the opportunity to learn a bit more. Bit by bit, bird by bird. That’s all we can do!
[date_published] => 2019-11-14T00:00:00-08:00
[url] => https://www.jwithy.com/promising-design-patterns
)
[7] => Array
(
[id] => https://www.jwithy.com/process
[slug] => process
[title] => Process
[summary] => I’m definitely a visual thinker/learner (close second to audio learning style), and I also love to map a thing out ahead of time so I don’t have to
[image] => https://www.jwithy.com/_thumbnails/ef0dc2e7-2961-4248-93d3-21a036319abe/large.jpg
[content_html] => I’m definitely a visual thinker/learner (close second to audio learning style), and I also love to map a thing out ahead of time so I don’t have to track it in my brain.
Sometimes that means that if I’m breaking things down into components that I make some wireframe-y sketches! to Here’s a couple of examples of how that looks, from a thing I worked on this weekend:
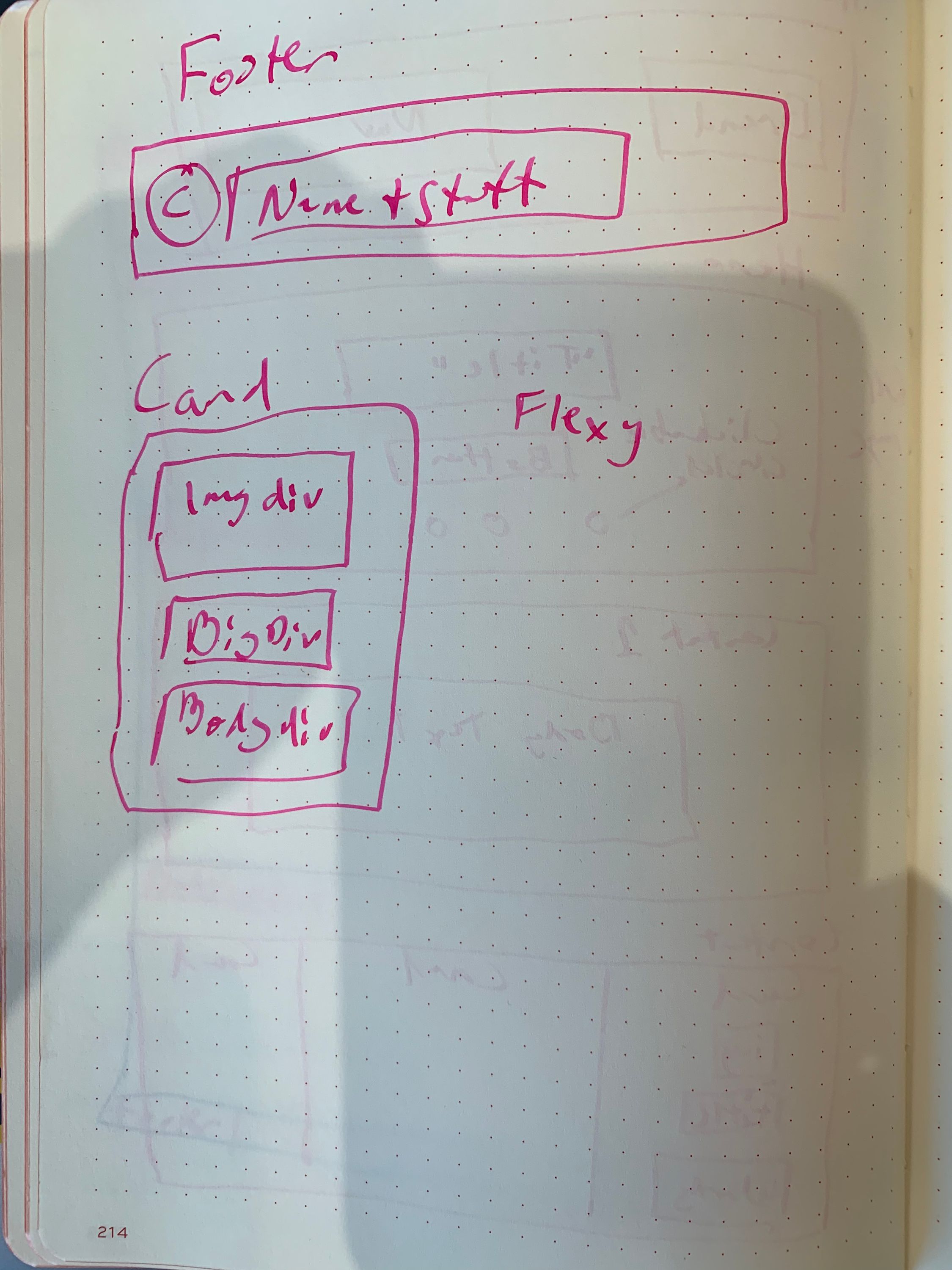
Bonus: I get to use my fancy fountain pens!
[date_published] => 2019-06-10T00:00:00-07:00
[url] => https://www.jwithy.com/process
)
[8] => Array
(
[id] => https://www.jwithy.com/whoa-imagemagick
[slug] => whoa-imagemagick
[title] => Whoa ImageMagick!
[summary] => Holy whoa this is cool: by using ImageMagick’s command line interface, you can combine images using terminal commands like:
convert +append a.png
[content_html] => Holy whoa this is cool: by using ImageMagick’s command line interface, you can combine images using terminal commands like:
convert +append a.png b.jpg c.tif
This is super cool if you don’t feel like fussing a ton with Preview.app, or worse, launching Photoshop, etc., OR if you need to convert to a different image type (note the different extensions, above).
I used it today becaue I had a few screenshots I wanted to combine and markup for posting in an pull request. Instead of having to do all that manually, I did this:
convert +append form1.png form2.png form3.png form-all.png
…and then marked up the new image (which was form-all.png
) by just by hitting spacebar in Finder and using Markup.
Easy Peasy!
I learned about ImageMagick by Googling combine three images preview mac osx
which led me to StackExchange).
I installed it with brew install imagemagick
; this led to an error message (Permission denied @ dir_s_mkdir - /usr/local/Frameworks
), which was resolved by Googling the Error message, which took me here.
Just a small glimpse into the way that anything involving web dev is time consuming. 😀
Happy combining!
[date_published] => 2019-05-28T00:00:00-07:00
[url] => https://www.jwithy.com/whoa-imagemagick
)
[9] => Array
(
[id] => https://www.jwithy.com/literally-speaking
[slug] => literally-speaking
[title] => Literally Speaking
[summary] => Yesterday I put in a pull request for the framework that we use at work. It was related to an issue I’d submitted; namely, that I wanted to be able
[content_html] => Yesterday I put in a pull request for the framework that we use at work. It was related to an issue I’d submitted; namely, that I wanted to be able to send folks style guide links to specific variants of components we build.
Building this had a lot of codey type things in it:
- Thinking about how to build it took longer than building it! This happens not infrequently, but it’s definitely surprising to newer folks.
- I played a bit with regular expressions. Regex is a weird thing, but I didn’t want to have spaces in URLs, since those can still cause problems. A bit of googling found me the expression I needed, but I also had to fully understand the JavaScript in order to do it.
- JavaScript! I’m working on learning a lot more JS; I think it’s a case of “I know more than I think I do,” but also, it took me a minute to figure out how to do this:
// for permalinks to examples
const exampleName = example.name;
const exampleNameNoSpace = exampleName.replace(/\s+/g, '');
const exampleLink = `#${exampleNameNoSpace}`;
However, I did it!
You see, when you look up examples for using these sorts of expressions, programmers like to use variables (like str
for string) that feel like they mean more than they do. So many examples used str.replace(/\s+/g
that I thought it meant something important. Nope! This was just convention, used by many folks, but not necessary.
And hey, it worked!
One of the main things I had to figure out is how .jsx — combined with the linting we use — would want me to combine the #
needed for an anchor link on the same page with the exampleNameNoSpace
variable I’d created above it.
These are “template literals,” and though I could have concatenated the two parts, ESLint wasn’t having it. The cool thing is that the linter’s warning taught me a new thing I needed to know.
So yeah! That’s what I learned yesterday. How bout you?
[date_published] => 2019-01-31T00:00:00-08:00
[url] => https://www.jwithy.com/literally-speaking
)
[10] => Array
(
[id] => https://www.jwithy.com/hello-world
[slug] => hello-world
[title] => Hello, World!
[summary] => This thing looks pretty swell. Let’s see how it goes!
[content_html] => This thing looks pretty swell. Let’s see how it goes!
[date_published] => 2017-12-27T09:00:35-08:00
[url] => https://www.jwithy.com/hello-world
)
)
)